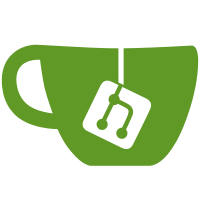
aebe4c0 Arduino Ant now works. d3cb4c1 Merge commit 'fbeed8e80922152c3404fbd5d2b243ae95792ec1' into V2 fbeed8e Used Client override for processing messages 394dc22 Merge commit '355dd5c1c519cf07cfb6b9f9200f7f7311e68f20' into V2 355dd5c Fixed ThingMsg format becb194 Merge commit 'f35d60369daf41a4fcd987ef8b31bd384b9536ba' into V2 9b53eee Merge commit 'a48ae12fc2f6d4a99119c128e78bf4b103e607c3' into V2 f35d603 Further improvements a48ae12 ControlCore mostly works (but I don't see a model on the site server yet) d8fc41f First step for ControlCore support git-subtree-dir: Runtime/HumanoidControl/Scripts/Networking/Roboid/ControlCore git-subtree-split: aebe4c0f8e805259a5aea4a4cb6b72343d73257a
109 lines
3.3 KiB
C#
109 lines
3.3 KiB
C#
using System.Collections.Generic;
|
|
using System.Collections.Concurrent;
|
|
using System.Net.Sockets;
|
|
|
|
namespace Passer.Control {
|
|
|
|
public class Client {
|
|
//public ConnectionMethod connection;
|
|
public UdpClient udpClient;
|
|
public string ipAddress;
|
|
public int port;
|
|
|
|
public byte networkId = 0;
|
|
|
|
public readonly ConcurrentQueue<IMessage> messageQueue = new();
|
|
|
|
public static Client GetClient(string ipAddress, int port) {
|
|
foreach (Client c in clients) {
|
|
if (c.ipAddress == ipAddress && c.port == port)
|
|
return c;
|
|
}
|
|
return null;
|
|
}
|
|
static public List<Client> clients = new List<Client>();
|
|
|
|
//// These static functions are deprecated
|
|
//public static Client NewClient() {
|
|
// Client client = new();
|
|
// clients.Add(client);
|
|
// client.networkId = 0;
|
|
|
|
// return client;
|
|
//}
|
|
|
|
//public static Client NewUDPClient(UdpClient udpClient, string ipAddress, int port) {
|
|
// Client client = NewClient();
|
|
// client.ipAddress = null;
|
|
// client.port = port;
|
|
// client.udpClient = udpClient;
|
|
// return client;
|
|
//}
|
|
|
|
//public Client() {
|
|
|
|
//}
|
|
|
|
public Client(UdpClient udpClient, int port) {
|
|
this.udpClient = udpClient;
|
|
this.ipAddress = null;
|
|
this.port = port;
|
|
clients.Add(this);
|
|
}
|
|
|
|
public void ProcessMessage(IMessage msg) {
|
|
switch (msg) {
|
|
case ClientMsg clientMsg:
|
|
ProcessClient(clientMsg);
|
|
break;
|
|
case NetworkIdMsg networkId:
|
|
ProcessNetworkId(networkId);
|
|
break;
|
|
case InvestigateMsg investigate:
|
|
ProcessInvestigate(investigate);
|
|
break;
|
|
case ThingMsg thing:
|
|
ProcessThing(thing);
|
|
break;
|
|
case NameMsg name:
|
|
ProcessName(name);
|
|
break;
|
|
case ModelUrlMsg modelUrl:
|
|
ProcessModelUrl(modelUrl);
|
|
break;
|
|
case PoseMsg pose:
|
|
ProcessPose(pose);
|
|
break;
|
|
case CustomMsg custom:
|
|
ProcessCustom(custom);
|
|
break;
|
|
case TextMsg text:
|
|
ProcessText(text);
|
|
break;
|
|
case DestroyMsg destroy:
|
|
ProcessDestroy(destroy);
|
|
break;
|
|
}
|
|
}
|
|
|
|
protected virtual void ProcessClient(ClientMsg client) { }
|
|
|
|
protected virtual void ProcessNetworkId(NetworkIdMsg networkId) { }
|
|
|
|
protected virtual void ProcessInvestigate(InvestigateMsg investigate) { }
|
|
|
|
protected virtual void ProcessThing(ThingMsg thing) { }
|
|
|
|
protected virtual void ProcessName(NameMsg name) { }
|
|
|
|
protected virtual void ProcessModelUrl(ModelUrlMsg modelUrl) { }
|
|
|
|
protected virtual void ProcessPose(PoseMsg pose) { }
|
|
|
|
protected virtual void ProcessCustom(CustomMsg custom) { }
|
|
|
|
protected virtual void ProcessText(TextMsg text) { }
|
|
|
|
protected virtual void ProcessDestroy(DestroyMsg destroy) { }
|
|
}
|
|
} |